Trigrep - Automated Security Scanning for Source Code with the Power of Semgrep and Trivy Integration
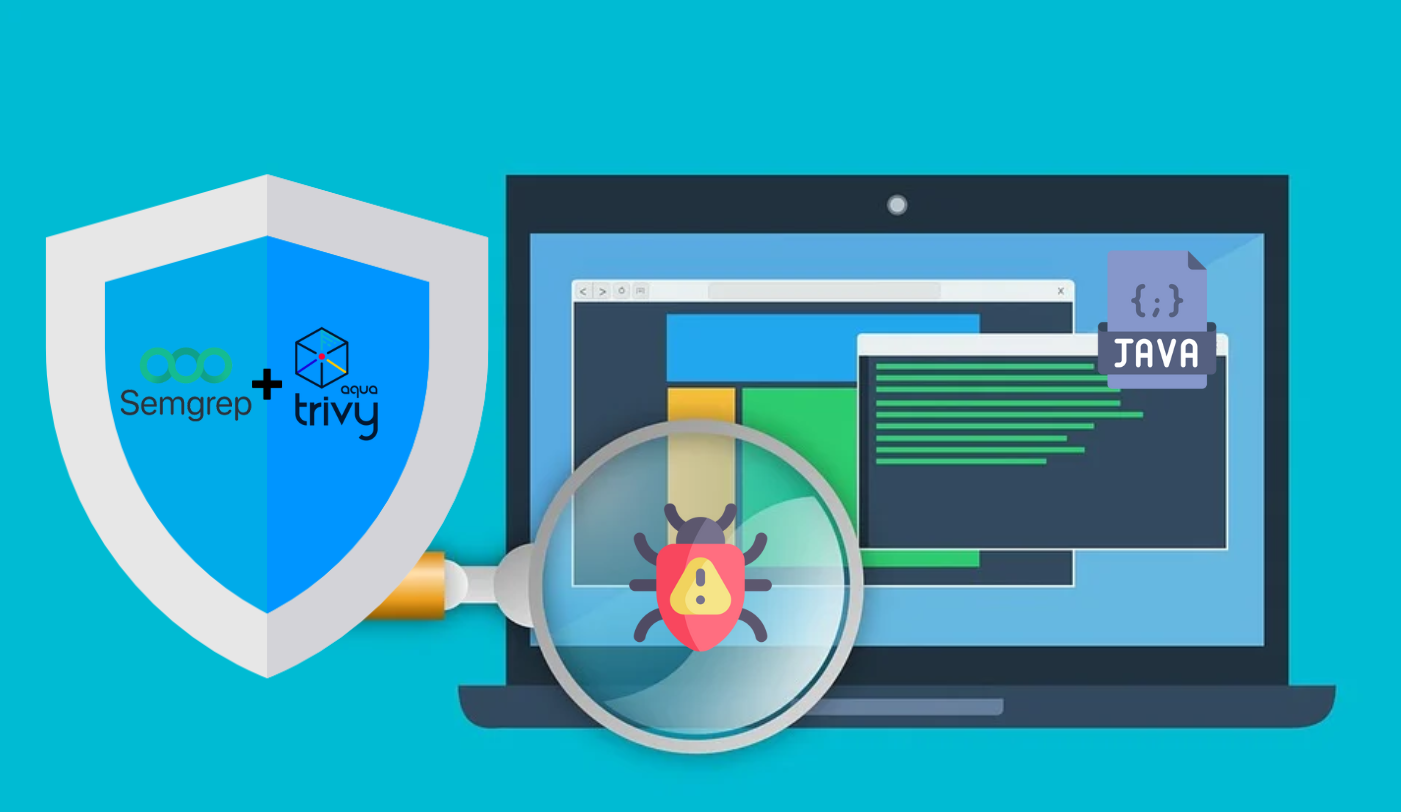
Understanding SAST and SCA
- SAST (Static Application Security Testing): This technique analyzes source code, bytecode, or binary code for vulnerabilities without executing the application. It is useful for detecting security issues such as injection flaws, insecure coding practices, and hardcoded credentials.
- SCA (Software Composition Analysis): SCA scans third-party dependencies and libraries for known vulnerabilities by checking them against public vulnerability databases like the National Vulnerability Database (NVD) and GitHub Advisory.
Trivy and Semgrep complement each other by covering both these testing methodologies.
Overview of Trivy and Semgrep
Trivy – A Powerful SCA and Container Security Scanner
Trivy is an open-source vulnerability scanner developed by Aqua Security that performs dependency analysis and security scanning for:
- Source code repositories
- Container images
- File systems
- Virtual machines
- Kubernetes clusters
Why Trivy
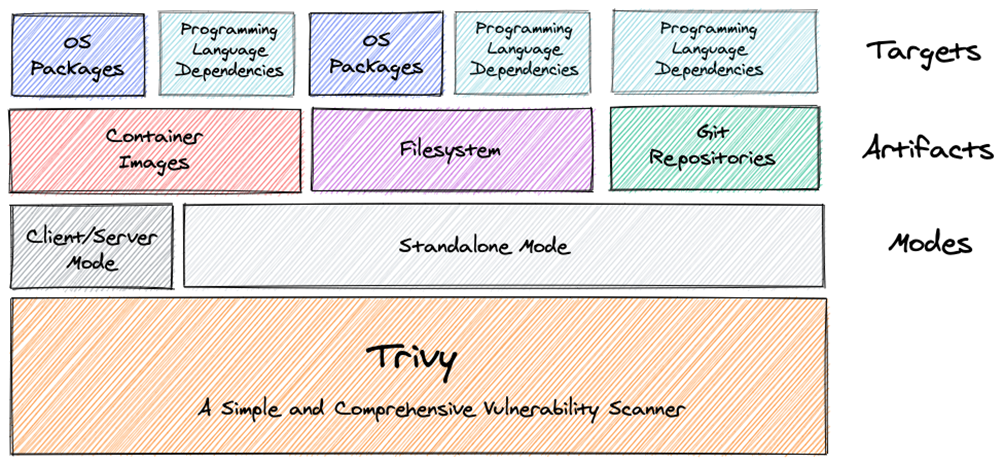
- Fast and efficient scanning with minimal configuration.
- Supports multiple formats (JSON, SARIF, table view) for easy integration with security dashboards.
- Extensive vulnerability database including NVD, GitHub Advisory Database, and more.
- Detects vulnerabilities in OS packages, dependencies (Maven, npm, Go modules, etc.), and misconfigurations.
Semgrep – A Lightweight and Customizable SAST Tool
Semgrep is a powerful static code analysis tool designed to detect security flaws in source code using pattern-based matching. It provides a highly customizable rule engine, making it a flexible alternative to traditional SAST solutions.
Why Semgrep
- Supports multiple programming languages including Java, JavaScript, Python, and Go.
- Lightweight and does not require compilation or deep program analysis.
- Allows developers to define custom security rules tailored to their application.
- Fast execution, making it ideal for CI/CD pipelines and DevSecOps workflows.
Integrating Trivy & Semgrep into a Web Application
To demonstrate the effectiveness of Trivy and Semgrep, I developed a Flask-based web application that centralizes security scanning results. The system allows users to:
- Upload their Java project.
- Execute scans using Trivy (for dependency analysis) and Semgrep (for source code analysis).
- View and manage security findings through an intuitive web dashboard.
Technology Stack
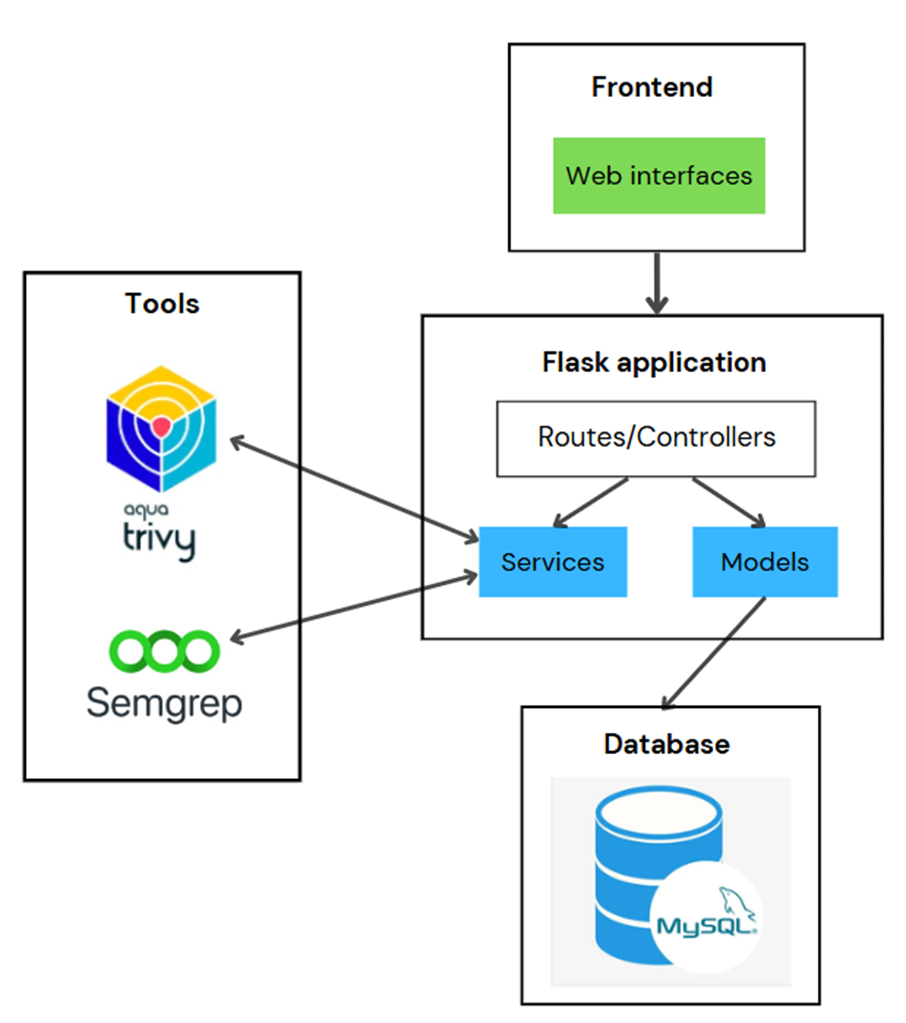
- Backend: Flask (Python)
- Database: MySQL with SQLAlchemy ORM
- Frontend: Bootstrap 5, jQuery, DataTables
- Security Tools: Trivy (CLI-based scanning), Semgrep (custom rule-based scanning)
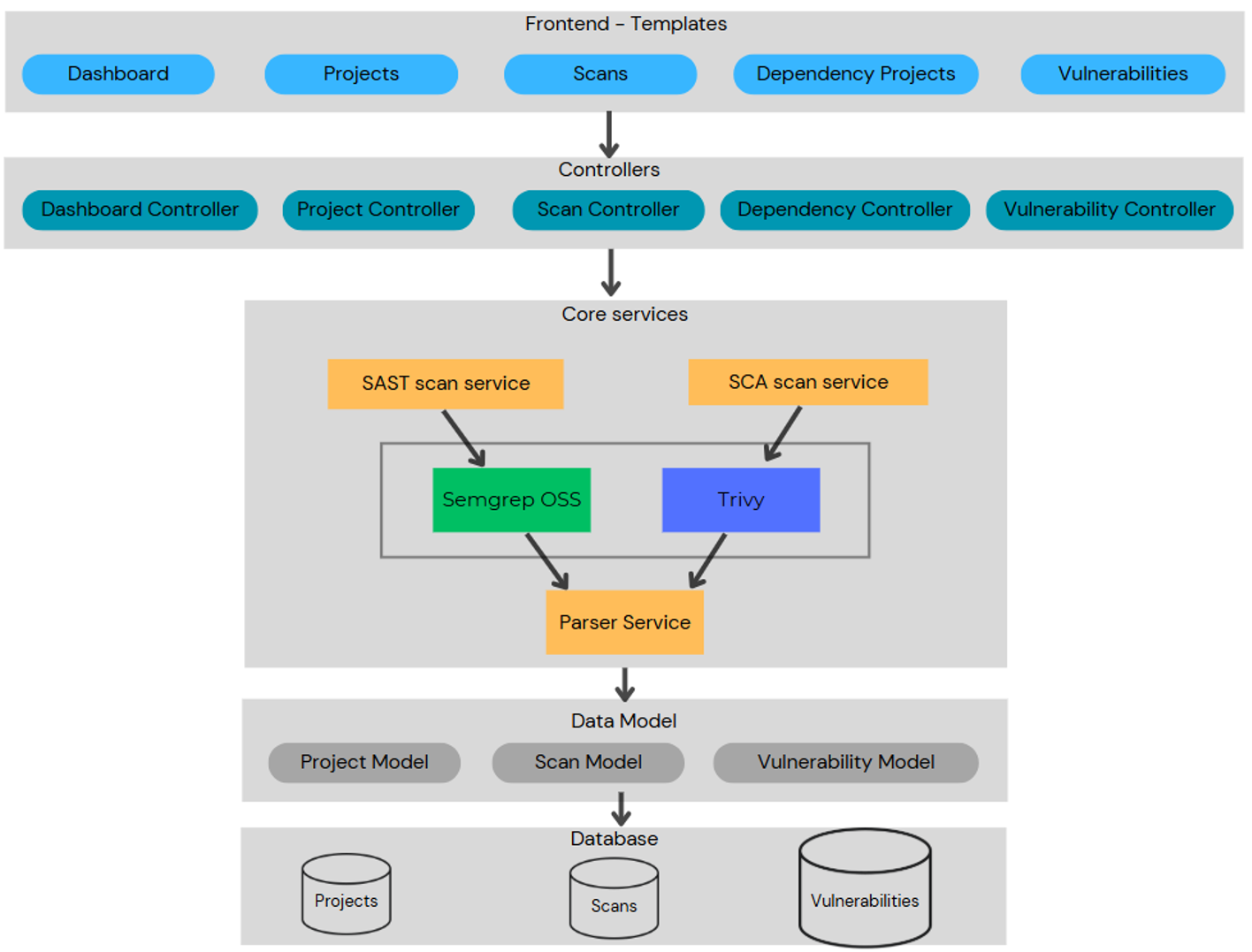
Demo
Insecure bank
I perform a scan on target:
- Android Application: insecure-bank v2
Access the website at: http://203.162.xx.xxx/login and log in with the username:password as 0xl4p:0xl4p@2025.
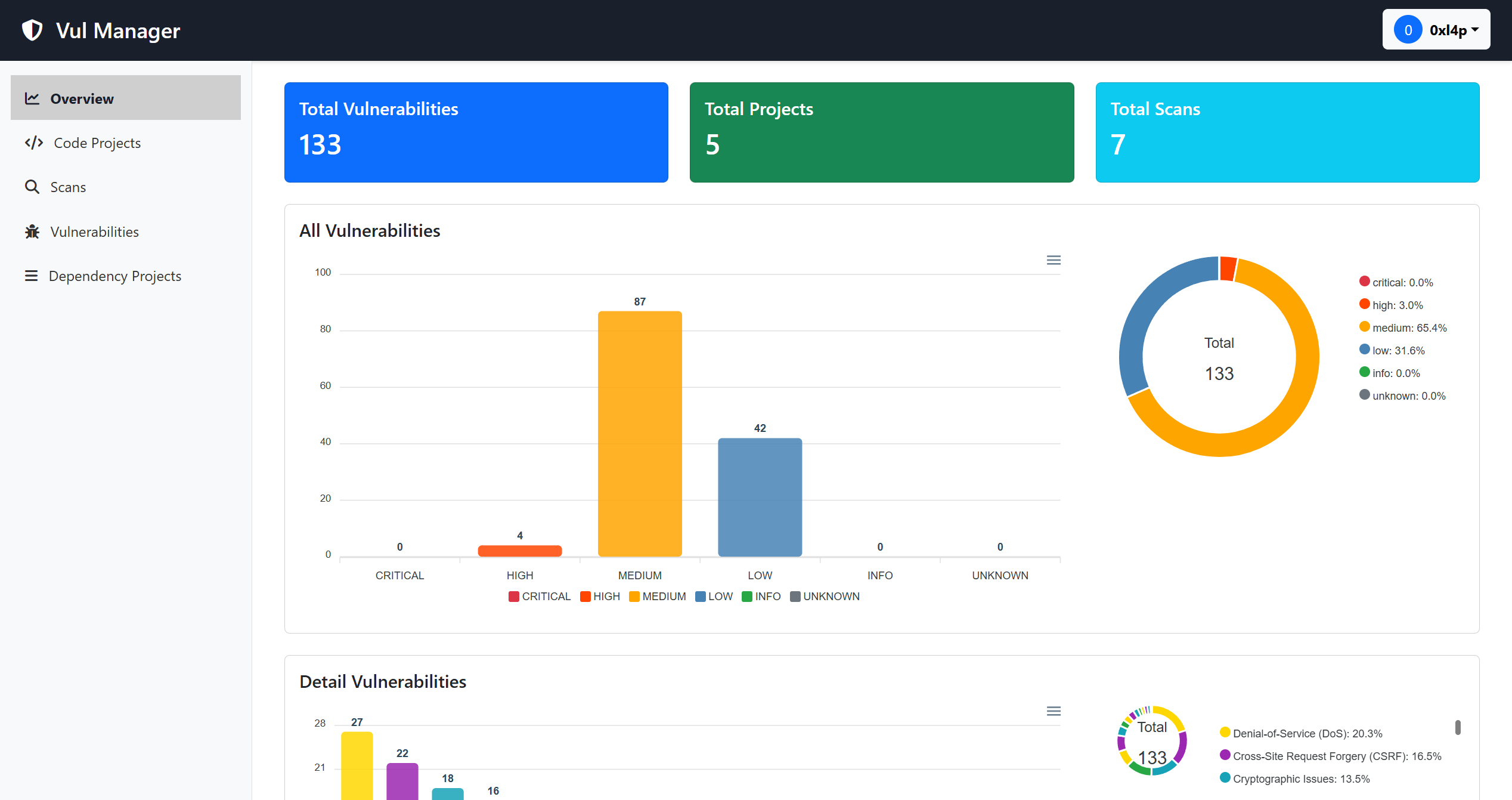
Create a new project "Insecure Bank v2" and upload the source code to the website
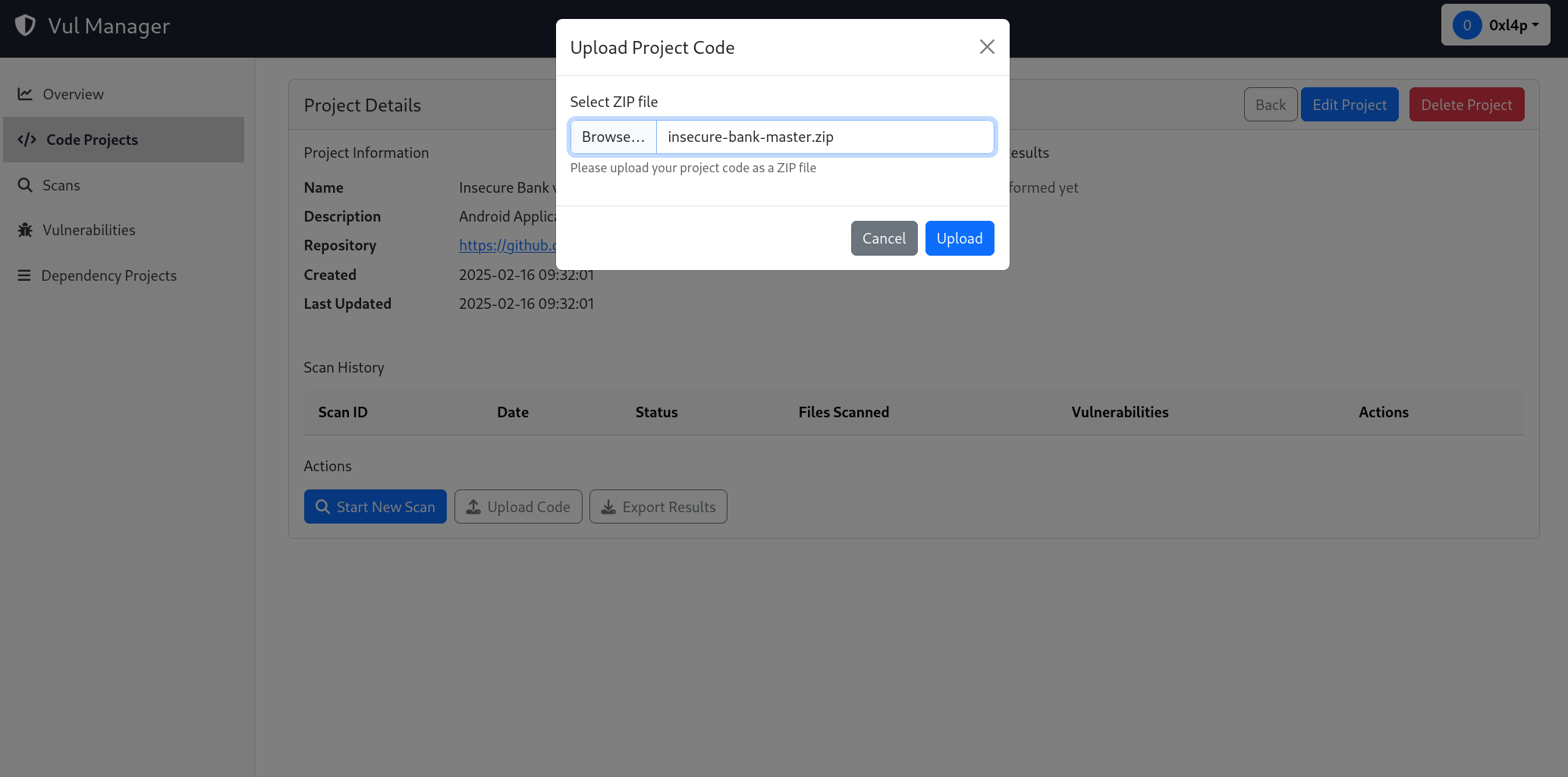
Select Scan Type, here I use SAST (ie Semgrep will be used in this case), on the contrary, Trivy will perform SCA.
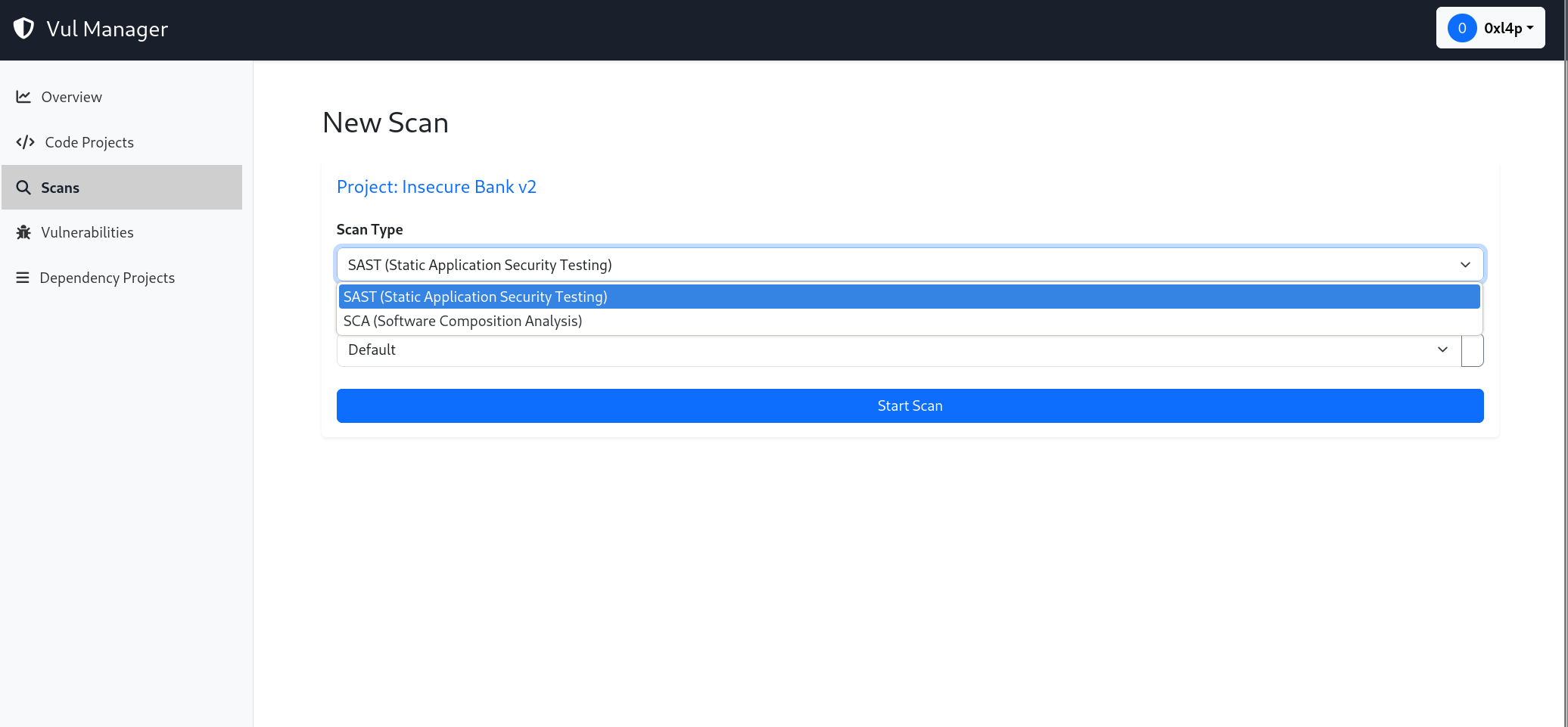
The results:
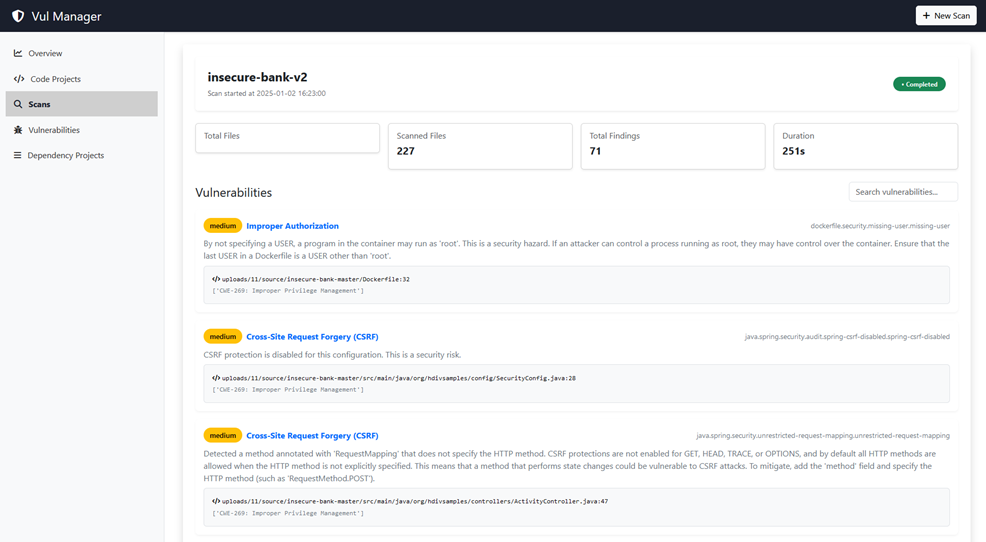
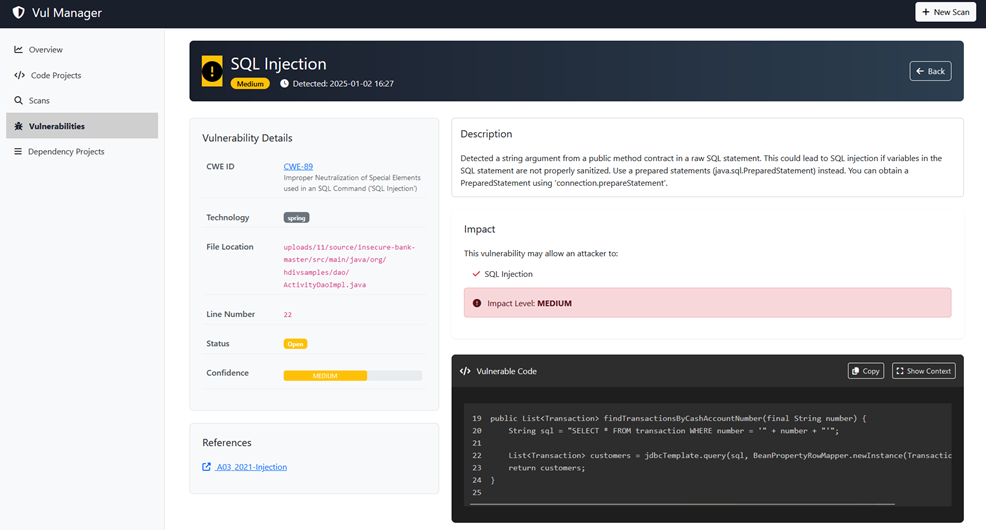
Select Scan type as SCA to scan for security vulnerabilities in the application's dependencies and libraries

CVE-2024-22243
The Open Redirect vulnerability (CVE-2024-22243) has been identified in Spring, allowing attackers to bypass URL authentication, leading to Open Redirect or SSRF attacks. This vulnerability occurs when UriComponentsBuilder is used to parse externally supplied URLs for server authentication validation.
- Product: Spring Framework
- Affected Packages:
spring-web
- Affected Versions:
>= 4.3.0 <= 4.3.30
>= 5.3.0 < 5.3.32
>= 6.0.0 < 6.0.17
>= 6.1.0 < 6.1.4
- GitHub Repository:
Reproduce
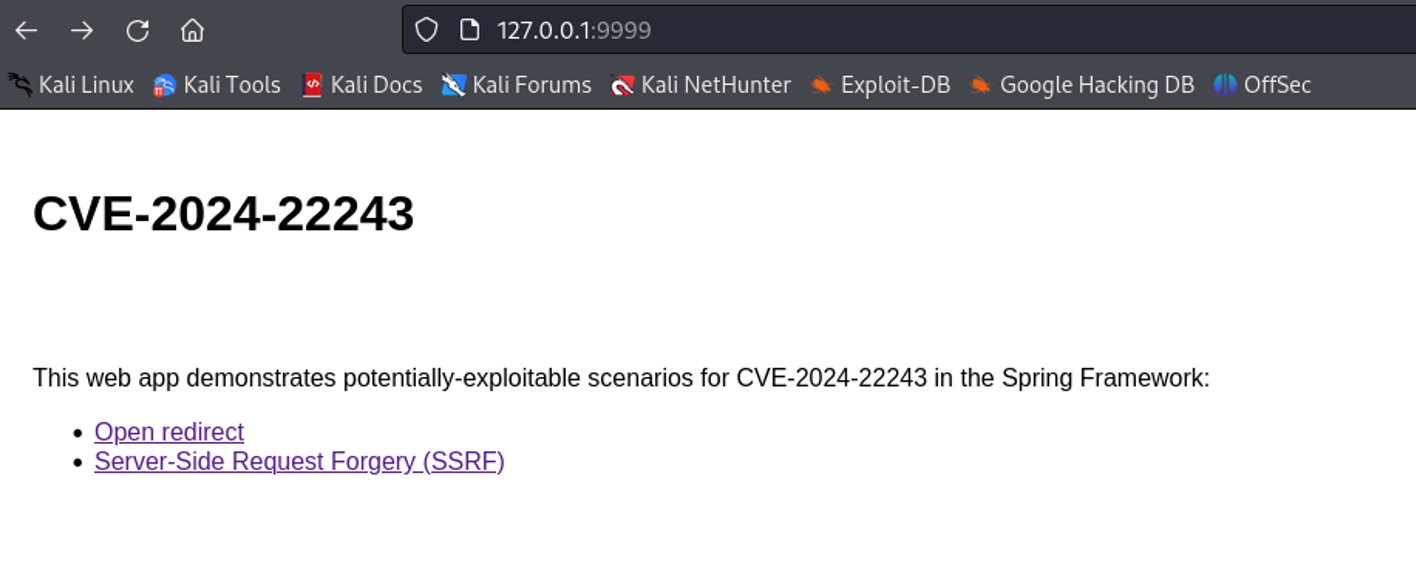
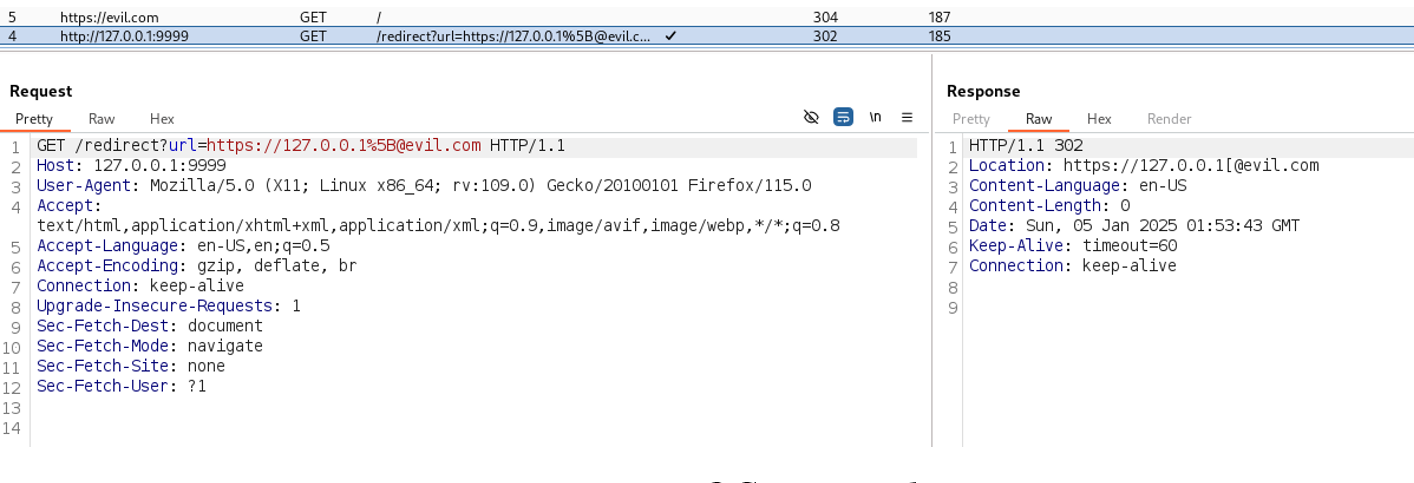
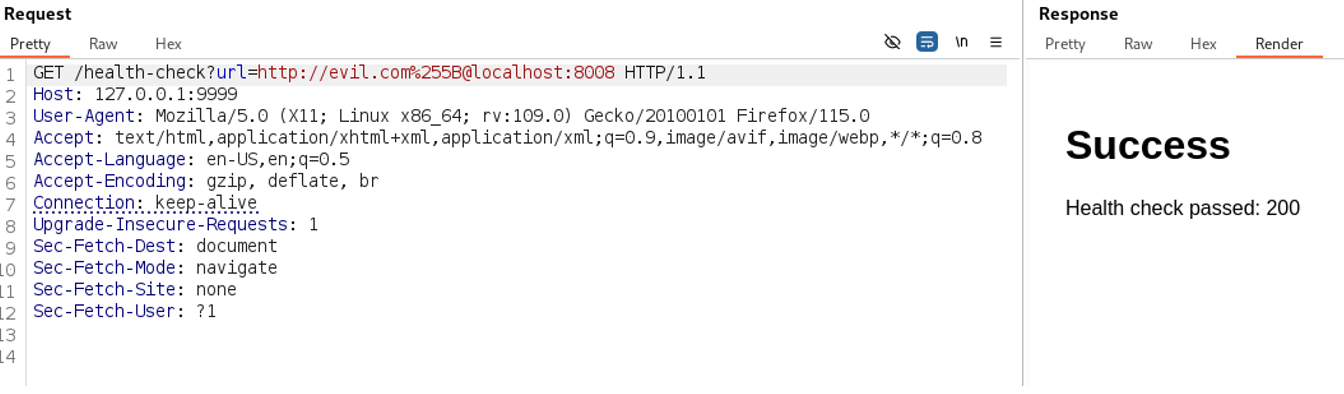
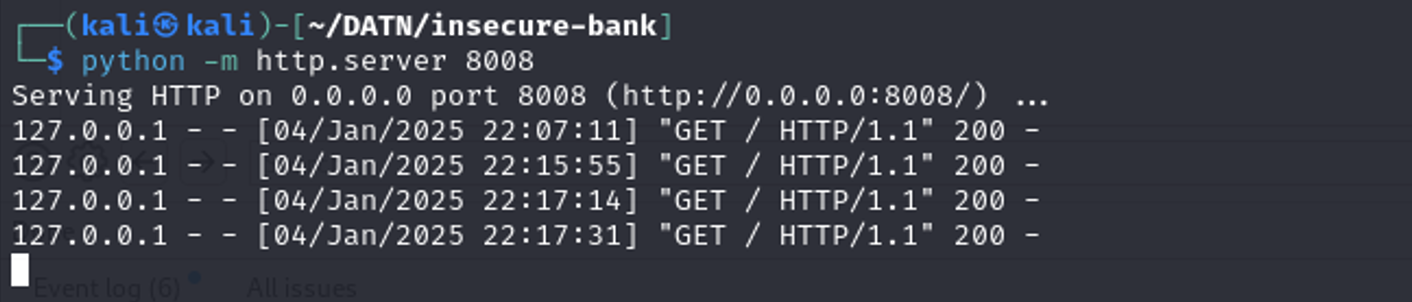
Custom Rules for Semgrep
Detecting unsafe use of Uricombonentsbuilder
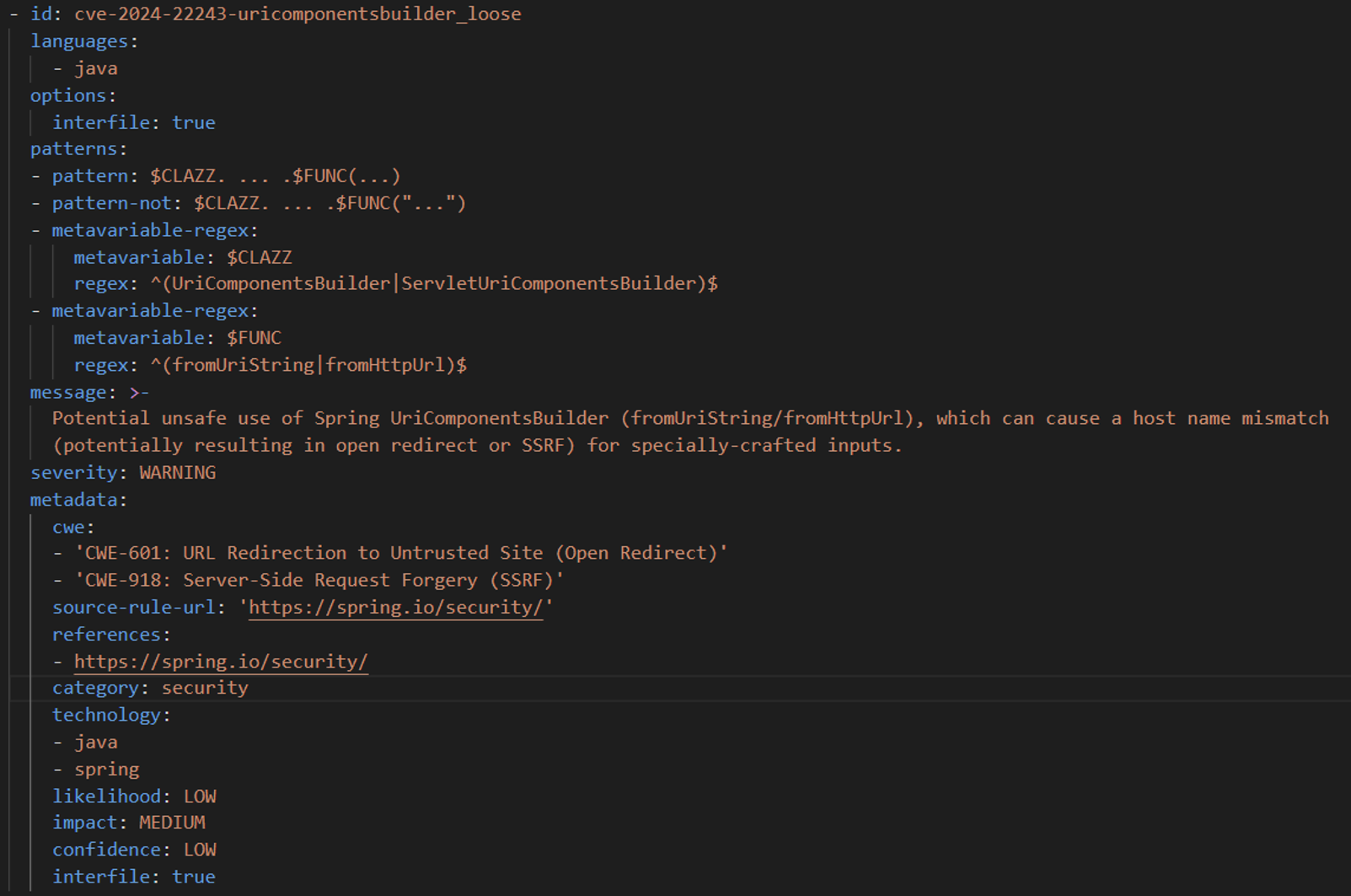
UriComponentsBuilder.fromUriString
Detecting unsafe use of RestTemplate
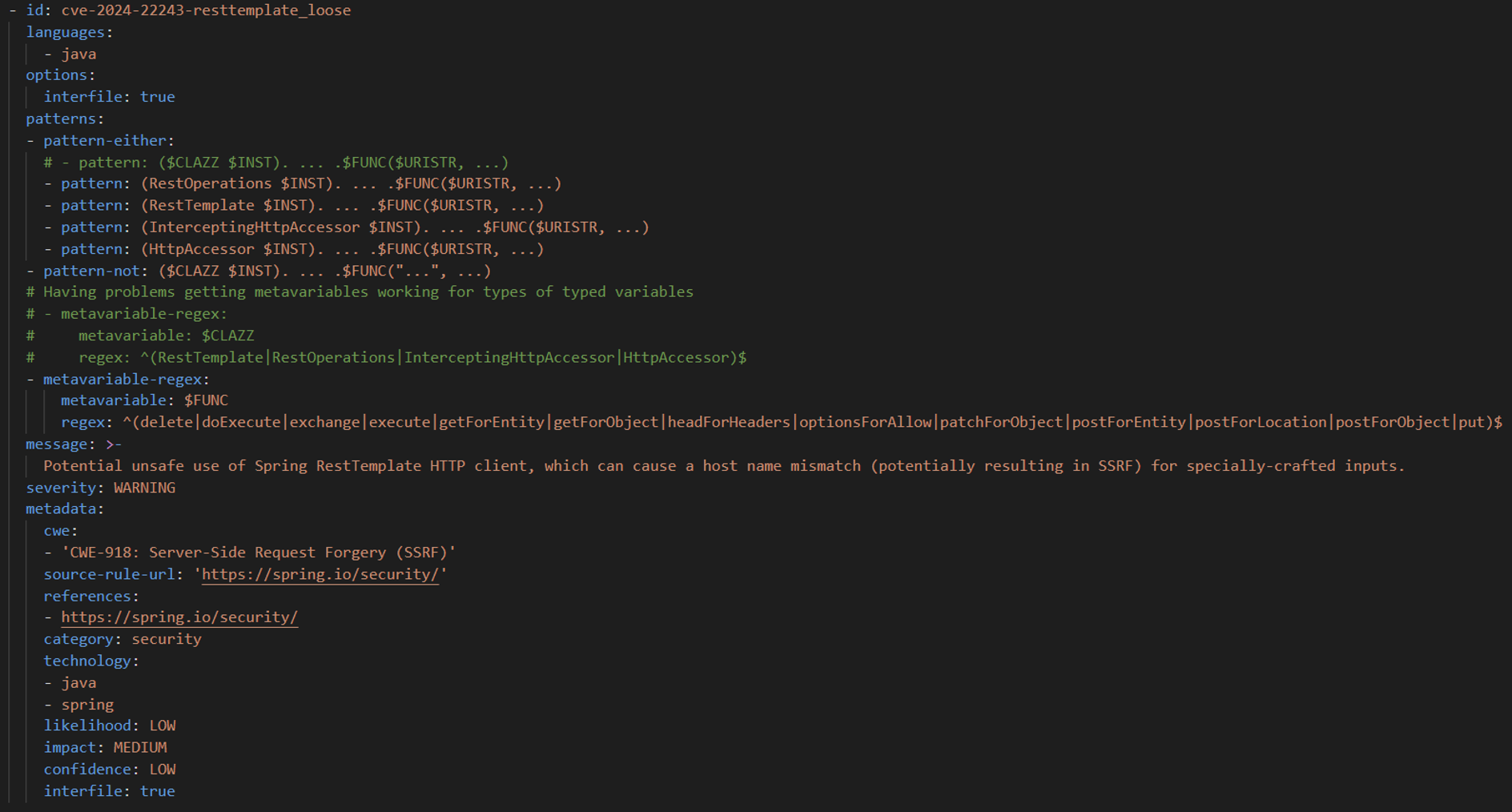
RestTemplate.exchange
Detecting unsafe use of WebClient and RestClient
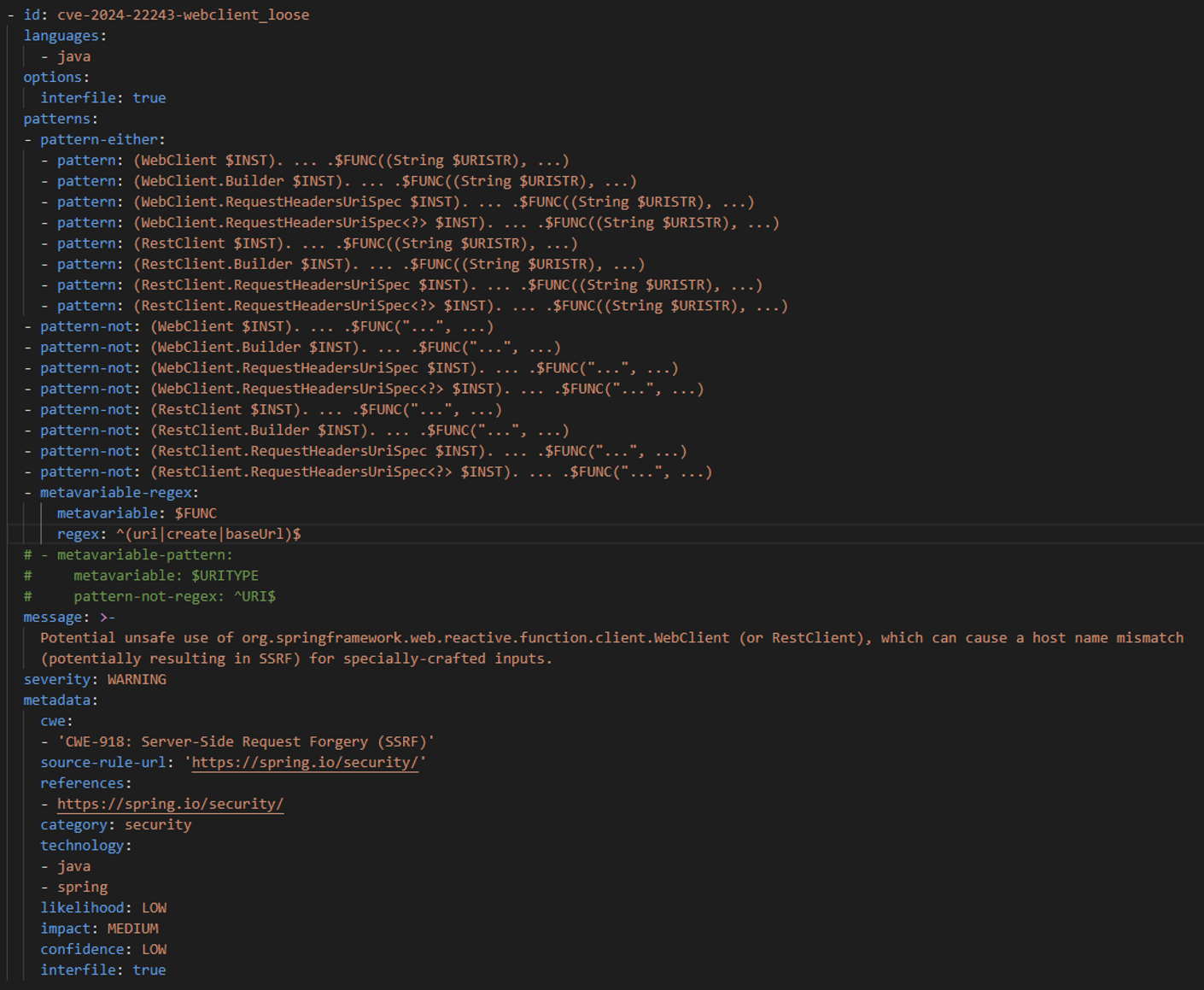
WebClient
Using Untrusted StringsCheck for the usage of UriComponentsBuilder
combined with getHost()
within the same scope
- id: cve-2024-22243-uricomponentsbuilder_strict
languages:
- Java
options:
interfile: true
patterns:
- pattern-either:
- patterns:
- pattern: $CLAZZ. ... .$FUNC(...). ... .getHost()
- pattern-not: $CLAZZ. ... .$FUNC("..."). ... .getHost()
- patterns:
- pattern: |
(UriComponents $INST) = $CLAZZ. ... .$FUNC(...). ... .build();
...
(UriComponents $INST).getHost();
- pattern-not: |
(UriComponents $INST) = $CLAZZ. ... .$FUNC("..."). ... .build();
...
(UriComponents $INST).getHost();
- patterns:
- pattern: |
$BUILDER = $CLAZZ. ... .$FUNC(...). ... ;
(UriComponents $INST) = ($CLAZZ $BUILDER). ... .build();
...
(UriComponents $INST).getHost();
- pattern-not: |
$BUILDER = $CLAZZ. ... .$FUNC("..."). ... ;
(UriComponents $INST) = ($CLAZZ $BUILDER). ... .build();
...
(UriComponents $INST).getHost();
- metavariable-regex:
metavariable: $CLAZZ
regex: ^(UriComponentsBuilder|ServletUriComponentsBuilder)$
- metavariable-regex:
metavariable: $FUNC
regex: ^(fromUriString|fromHttpUrl)$
message: >-
Potential unsafe use of Spring UriComponentsBuilder (fromUriString/fromHttpUrl), which can cause a host name mismatch (potentially resulting in open redirect or SSRF) for specially-crafted inputs.
severity: WARNING
metadata:
cwe:
- 'CWE-601: URL Redirection to Untrusted Site (Open Redirect)'
- 'CWE-918: Server-Side Request Forgery (SSRF)'
source-rule-url: 'https://spring.io/security/'
references:
- https://spring.io/security/
category: security
technology:
- Java
- spring
likelihood: MEDIUM
impact: MEDIUM
confidence: MEDIUM
interfile: true
Taint flow analysis from user Input to Uricomponentsbuilder
- id: cve-2024-22243-uricomponentsbuilder_taint
languages:
- Java
options:
interfile: true
mode: taint
pattern-sources:
- patterns:
- pattern-either:
- patterns:
- pattern-either:
- pattern-inside: |
$METHODNAME(..., @$REQ(...) $TYPE $SOURCE, ...) {
...
}
- pattern-inside: |
$METHODNAME(..., @$REQ $TYPE $SOURCE, ...) {
...
}
- metavariable-regex:
metavariable: $TYPE
regex: ^(?!(Integer|Long|Float|Double|Char|Boolean|int|long|float|double|char|boolean))
- metavariable-regex:
metavariable: $REQ
regex: (RequestBody|PathVariable|RequestParam|RequestHeader|CookieValue|ModelAttribute)
- patterns:
- pattern-either:
- pattern-inside: |
@$ATTR
public $METHODRETTYPE $METHODNAME(..., $TYPE $SOURCE, ...) {
...
}
- pattern-inside: |
@$ATTR(...)
public $METHODRETTYPE $METHODNAME(..., $TYPE $SOURCE, ...) {
...
}
- metavariable-regex:
metavariable: $TYPE
regex: ^(?!(Integer|Long|Float|Double|Char|Boolean|int|long|float|double|char|boolean))
- metavariable-regex:
metavariable: $ATTR
regex: (Request|Delete|Get|Patch|Put|Post)Mapping
- focus-metavariable: $SOURCE
# Unclear whether these are helpful
pattern-propagators:
- pattern: $N = $CLASS. ... .$FUNC(..., $S, ...)
from: $S
to: $N
- pattern: $N = $S. ... .$FUNC(...)
from: $S
to: $N
pattern-sinks:
- patterns:
- pattern-either:
- patterns:
- pattern: $CLASS.$FUNC(...)
- metavariable-regex:
metavariable: $FUNC
regex: ^(fromUriString|fromHttpUrl)$
message: >-
Potential unsafe use of Spring UriComponentsBuilder (fromUriString/fromHttpUrl), which can cause a host name mismatch (potentially resulting in open redirect or SSRF) for specially-crafted inputs.
severity: WARNING
metadata:
cwe:
- 'CWE-601: URL Redirection to Untrusted Site (Open Redirect)'
- 'CWE-918: Server-Side Request Forgery (SSRF)'
source-rule-url: 'https://spring.io/security/'
references:
- https://spring.io/security/
category: security
technology:
- Java
- spring
likelihood: MEDIUM
impact: MEDIUM
confidence: MEDIUM
interfile: true
Taint flow analysis for WebClient/RestClient
- id: cve-2024-todo-webclient_taint
languages:
- Java
options:
interfile: true
mode: taint
pattern-sources:
- patterns:
- pattern-either:
- patterns:
- pattern-either:
- pattern-inside: |
$METHODNAME(..., @$REQ(...) $TYPE $SOURCE, ...) {
...
}
- pattern-inside: |
$METHODNAME(..., @$REQ $TYPE $SOURCE, ...) {
...
}
- metavariable-regex:
metavariable: $TYPE
regex: ^(?!(Integer|Long|Float|Double|Char|Boolean|int|long|float|double|char|boolean))
- metavariable-regex:
metavariable: $REQ
regex: (RequestBody|PathVariable|RequestParam|RequestHeader|CookieValue|ModelAttribute)
- patterns:
- pattern-either:
- pattern-inside: |
@$ATTR
public $METHODRETTYPE $METHODNAME(..., $TYPE $SOURCE, ...) {
...
}
- pattern-inside: |
@$ATTR(...)
public $METHODRETTYPE $METHODNAME(..., $TYPE $SOURCE, ...) {
...
}
- metavariable-regex:
metavariable: $TYPE
regex: ^(?!(Integer|Long|Float|Double|Char|Boolean|int|long|float|double|char|boolean))
- metavariable-regex:
metavariable: $ATTR
regex: (Request|Delete|Get|Patch|Put|Post)Mapping
- focus-metavariable: $SOURCE
# Unclear whether these are helpful
pattern-propagators:
- pattern: $N = $CLASS. ... .$FUNC(..., $S, ...)
from: $S
to: $N
- pattern: $N = $S. ... .$FUNC(...)
from: $S
to: $N
pattern-sinks:
- patterns:
- pattern-either:
- pattern: (WebClient $INST). ... .$SINKFUNC($SINK, ...)
- pattern: (WebClient.Builder $INST). ... .$SINKFUNC($SINK, ...)
- pattern: (WebClient.RequestHeadersUriSpec $INST). ... .$SINKFUNC($SINK, ...)
- pattern: (WebClient.RequestHeadersUriSpec<?> $INST). ... .$SINKFUNC($SINK, ...)
- pattern: (RestClient $INST). ... .$SINKFUNC($SINK, ...)
- pattern: (RestClient.Builder $INST). ... .$SINKFUNC($SINK, ...)
- pattern: (RestClient.RequestHeadersUriSpec $INST). ... .$SINKFUNC($SINK, ...)
- pattern: (RestClient.RequestHeadersUriSpec<?> $INST). ... .$SINKFUNC($SINK, ...)
- metavariable-regex:
metavariable: $SINKFUNC
regex: ^(uri|create|baseUrl)$
# - focus-metavariable: $SINK
message: >-
Potential unsafe use of org.springframework.web.reactive.function.client.WebClient (or RestClient), which can cause a host name mismatch (potentially resulting in SSRF) for specially-crafted inputs.
severity: WARNING
metadata:
cwe:
- 'CWE-918: Server-Side Request Forgery (SSRF)'
source-rule-url: 'https://spring.io/security/'
references:
- https://spring.io/security/
category: security
technology:
- Java
- spring
likelihood: MEDIUM
impact: MEDIUM
confidence: MEDIUM
interfile: true
I imported these rules to Semgrep and implemented it on web interface.
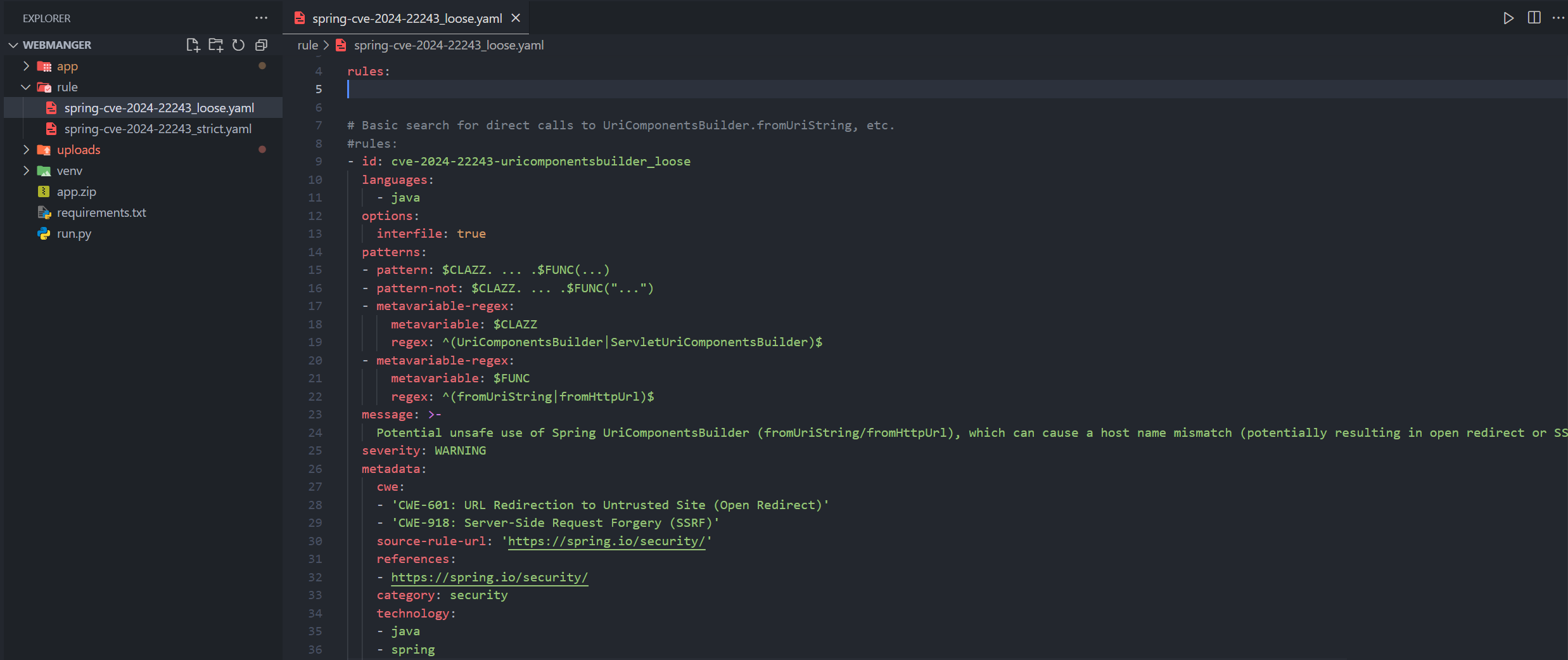
Scans
The scan results from Trivy indicate that the current Spring version of the application is affected by CVE-2024-22243.
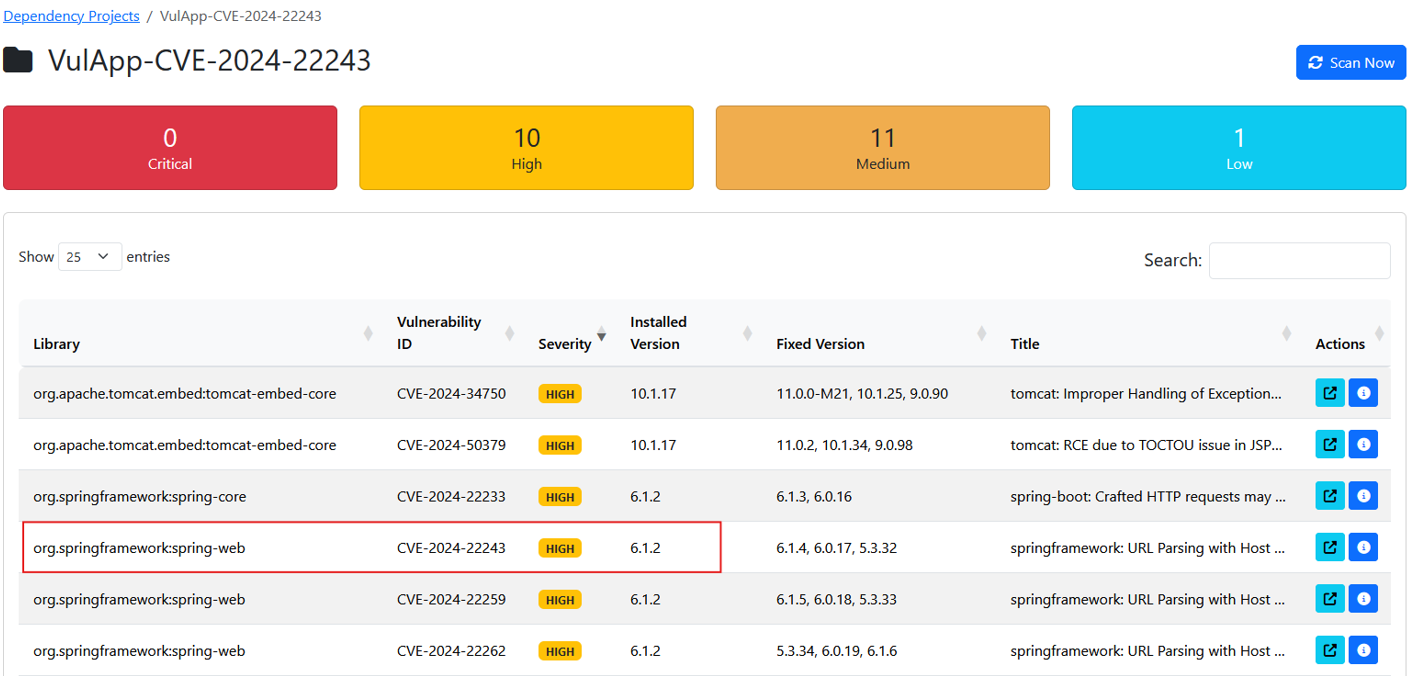
Perform a SAST scan with custom rules to assess the potential for CVE-2024-22243 vulnerabilities in the application's source code.
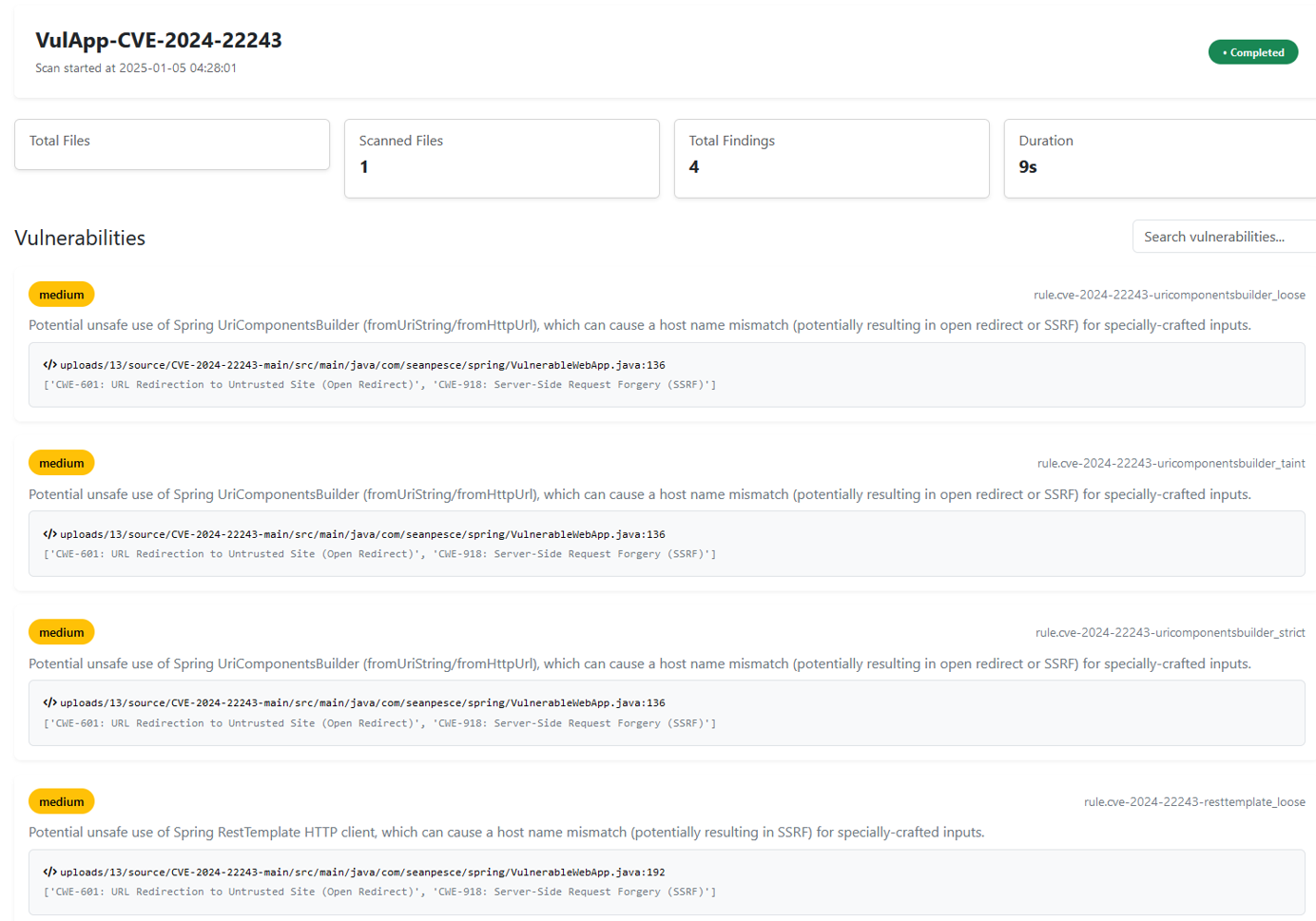
Resources:
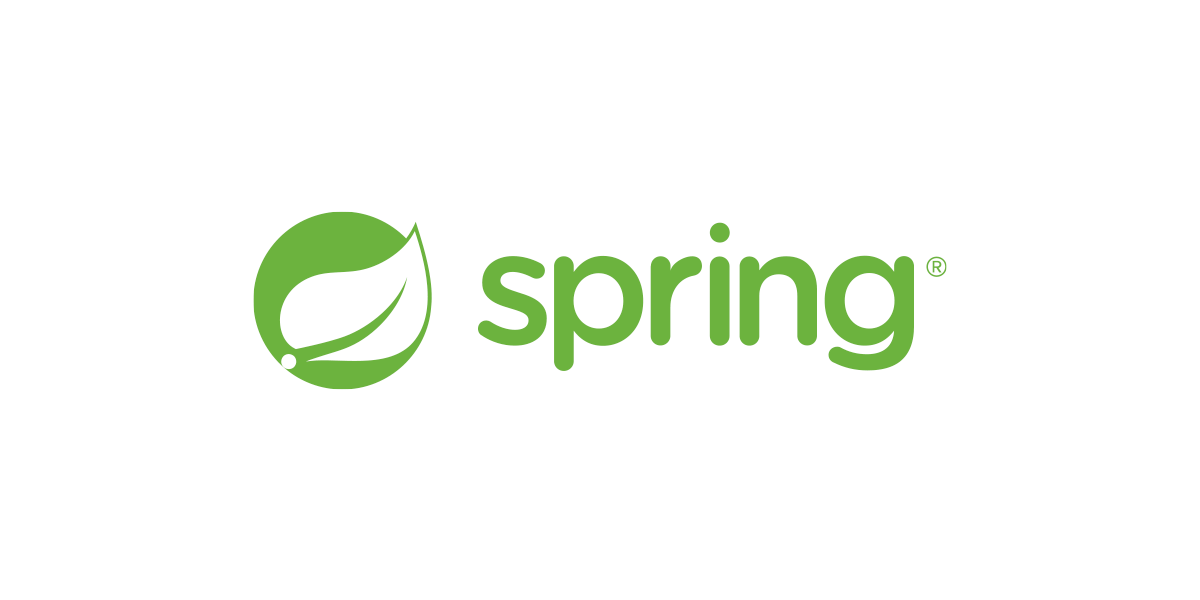